A trap: powershell variable is case insensitive
I have written a simple ps script to split a string and then loop from the output array. I tried to make it work for several days but it failed always. After tons of retries, I finally find out that ps variable name is case insensitive so my script cannot work as expected. And most importantly, the type of a variable can be changed or automatically inferred on the fly if you don’t specify a type at declaration. Let’s see below examples.
Example 1: auto variable type inference
function f($Tables) {
echo $Tables.GetType()
$tables = $Tables -Split ","
echo $tables.GetType()
foreach ($i in $tables) {
echo $i
}
}
f "a,b,c"
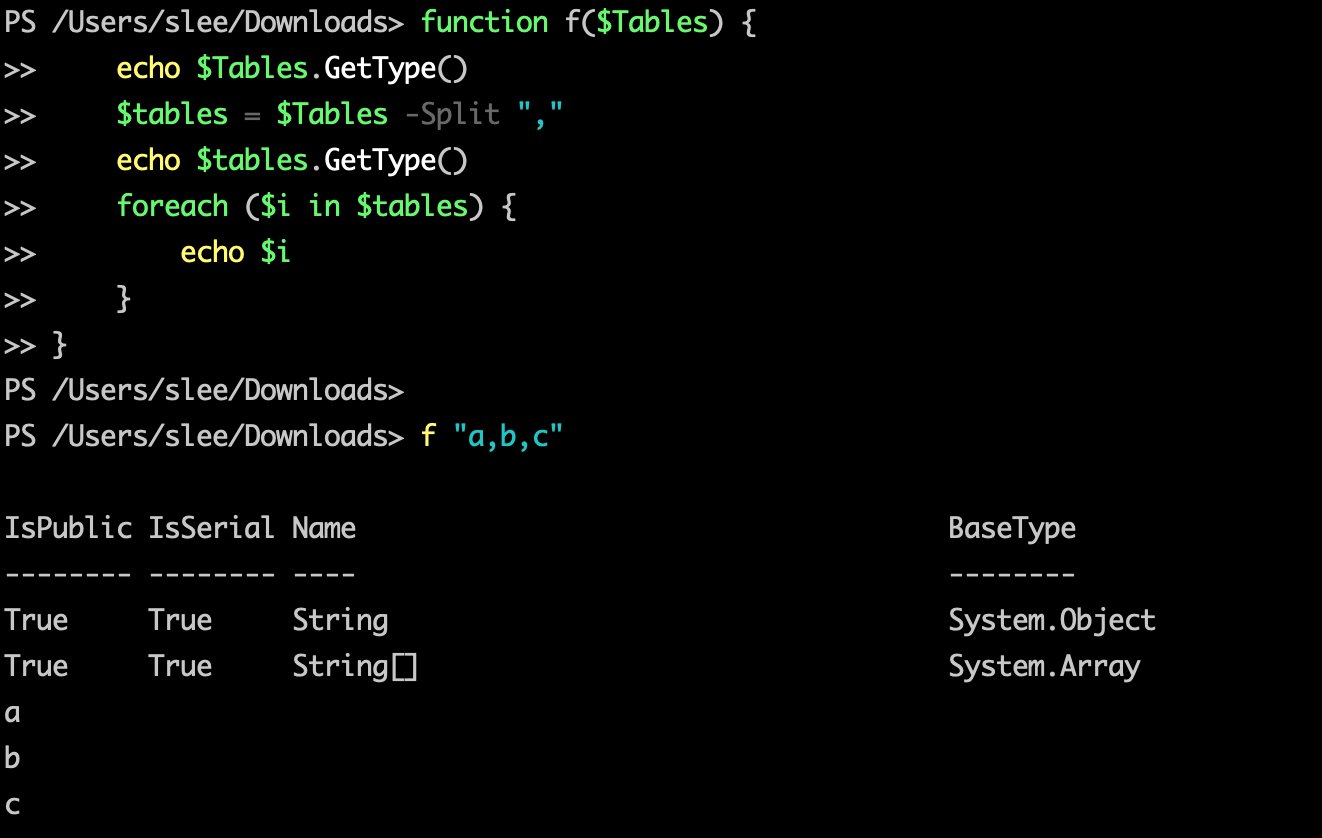
From the code output you can see that the type of Tables and tables are different. One is String and another is String array. That’s expected.
Example 2: explicit variable type declaration
function f([String]$Tables) {
echo $Tables.GetType()
$tables = $Tables -Split ","
echo $tables.GetType()
foreach ($i in $tables) {
echo $i
}
}
f "a,b,c"
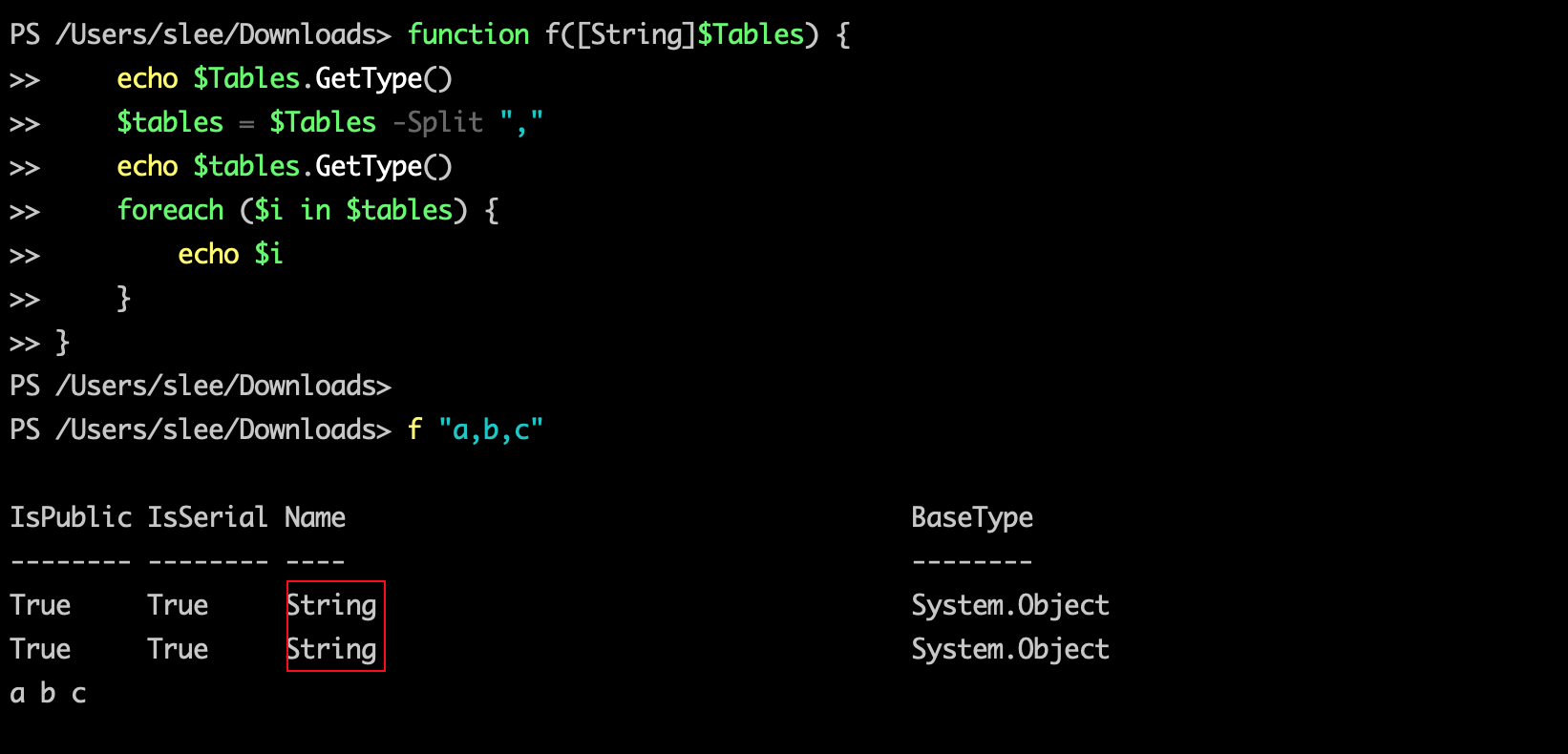
In this example, the type of Tables and tables are both String which is terrible. I don’t recognize the ps type system is not that strict until today. So it will be my habit to test a variable’s type through GeType() function in the following days.
WIL: variable name is case insensitive
$Tables = "a,b,c"
$tables # same output
$Tables # same output
"tables" -eq "Tables" # true, comparison operator is also case insensitvie
WIL: automatically type inference and type can be changed on the fly
$Tables = "a,b,c"
$Tables.GetType() # Sting
$Tables = $Tables -Split ","
$Tables.GetType() # String []
$a = "1"
2 * $a # output: 2, $a is converted to int when calculation